Today’s topic is Code Quality & Performance with Xamarin / Xamarin.Forms.
When we talk about code quality and performance we talk about the user’s experience. When our application is poor in performance can cause slow interactions with the users, our app can be freeze sometimes, our app can reduce battery life or the device can get hot just because of an operation in a loop that’s never ended.
However, when we talk about quality and performance there is more than just implementing efficient code. We can improve our application performance in many ways. There are many techniques, tons of articles, and some really nice conferences talking about this topic.
What I have here is a recompilation of all of them with some best practices, tools, techniques, and so on. I’m telling you, this article is going to blow your mind if you work with Xamarin.
What is Xamarin?
If your new in this world, just let’s start talking about what is XAMARIN. Xamarin is an open-source platform for building modern and performant applications for iOS, Android, and Windows with .NET. Xamarin is an abstraction layer that manages the communication of shared code with underlying platform code.
Xamarain Traditional
When we talk about Xamarin we have two approaches that we can follow. One of them is Xamarin Traditional (Or Xamarin Classic as some people call it).
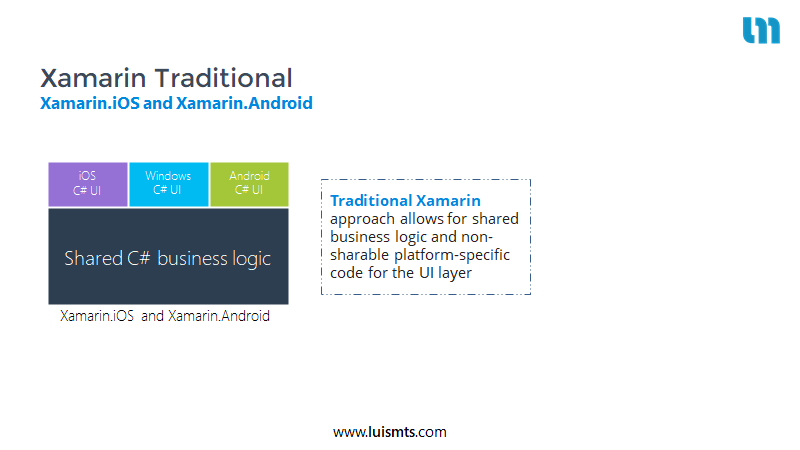
With this approach, you can write code logic once and share it across your Xamarin Android, iOS, and Windows projects, and in those separate projects you are writing code that is specific for that platform and you have access to their native APIs.
This means:
Anything you can do in Objective-C, Swift, or Java can be done in C# with Xamarin using Visual Studio, Visual Studio for Mac, and Visual Studio Code in near future with MAUI.
Xamarin.Forms
On the other hand Xamarin.Forms is a UI framework that allows developers to build Xamarin.iOS, Xamarin.Android, and Windows applications from a single shared codebase.
Performance
Brand new app?
The first thing is making good architecture choices.
- So pick a good MVVM framework like Prism, ReactiveUI, MvvmLight, or just what you like.
- If there are going to be databases involved, make sure you’re picking a good repository pattern.
If you’re new in the Xamarin Forms world or you have a small project Xamarin.Forms Shell can be a good option because you will have probably all the tools you need to work with.
Pick your dependencies wisely. So don’t go too dependency-heavy. Try to make your app light and snappy. So pick those wisely, and of course test often.
Already have an app?
The architecture that you have is the best choice? If it’s not I wrote an ebook with 7 Tactics to structure your Projects with Xamarin.Forms that you can use today for both new projects and projects already created.
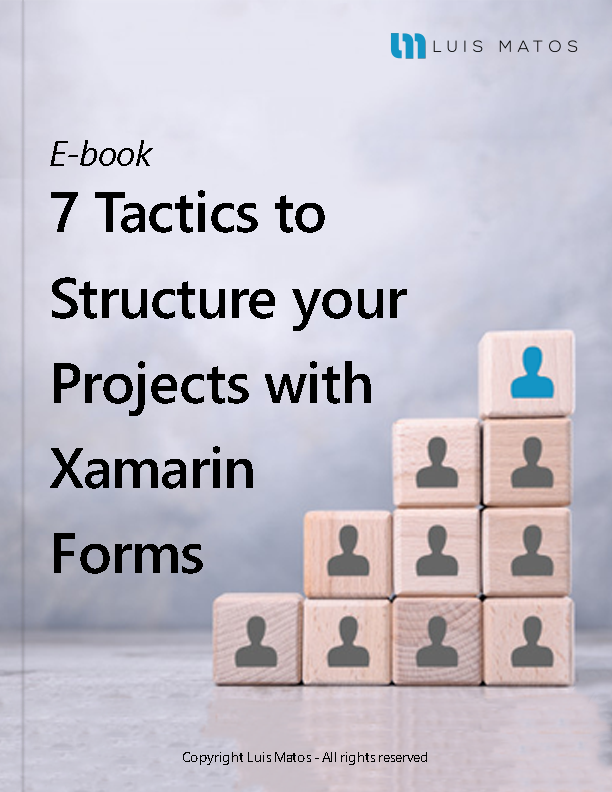
You can download here.
In the official Microsoft Xamarin Documentation, there is a section focus on improving Xamarin.Forms app performance.
Here some recommendation:
- Enable the XAML compiler*, Use compiled bindings*, Use fast renderers*. These options are enabled by default but for older solutions, you need to enable them manually.
- Reduce unnecessary bindings: Don’t use bindings for content that can easily be set statically. (For example the name of the page)
- Choose the correct layout: For example, if you have a stack layout, that is capable of having multiples children, with a single child is wasteful.
- Optimize layout performance, Use asynchronous programming and Choose a dependency injection container carefully are specific tips that I recommend you to follow in the guide.
- Use CollectionView instead of ListView: CollectionView is more flexible, and performant than ListView.
- If you are using ListView be sure to Optimize performance with Initialization, Scrolling, and Interaction.
- Reduce the visual tree size: Reducing the number of elements on a page will make the page render faster.
- Reduce the application resource dictionary size: XAML that’s specific to a page shouldn’t be included in the application’s resource dictionary, because the resources will be parsed at application startup instead of when is required by a page.
You can follow the complete guide here.
Creating apps with Xamarin.Forms
I wrote an article talking about Examples, and tips for creating applications in Xamarin Forms
In this article, I talk about The three pillars to develop better applications that are:
- Your app
- The purpose of your app and
- The developer
Also, I gave you some general tips and tips for increasing your application’s performance. In the performance section, I gave you some resources you can check.
An important tip:
Try to avoid RelativeLayout and AbsoluteLayout as much as you can.
Also in the same article, I gave some tips for Visual Studio and some tips to help you choose the best MVVM framework.
In the same way, you have their tips for your XAML and C# projects and much more.
You can read the complete article here.
Startup Performance
I know this is list is small but if you think about it I promise you, you will see the results immediately.
- Don’t start/register all services right in the beginning, lazyload where possible.
- Don’t download all your data on startup.
- Lazy Load Anything You Don’t Need Immediately.
- Think about User Experience.
Release configuration
Android
- Enable layout compression
- Enable startup tracing on Android
- Use AOT with startup tracing for faster startup.
- You can use custom profiles with startup tracing.
- Linker settings
- Turn on Link SDK Assemblies Only when your app is in Release [at the very least]
- Turn on Link All/SDK and User Assembles when your app is in Release [test manually]
- Enable [assembly:LinkerSafe] in binding projects
- Use Android App Bundles.
Here some resources:
- Optimize Xamarin.Android builds. link
- Xamarin Android App Bundles. link
- Xamarin Show – Android App Bundles. link
- David Ortinau’s Blog Post to boot Xamarin.Forms startup time. link
- Using Custom AOT Profiles with Xamarin.Android. link
- Faster Startup Times with Startup Tracing on Android. link
- Faster Application Startup using Custom Profiles with Startup Tracing. link
Linker resouces:
- Investing Time in the Xamarin Linker for Smaller App Sizes. link
- Optimizing Xamarin Apps & Libraries with the Linker. link
- Linking on Xamarin.Android. link
- Xamarin.Android Linker Tricks Part 1. link
iOS
- Enable LLVM optimizing compiler
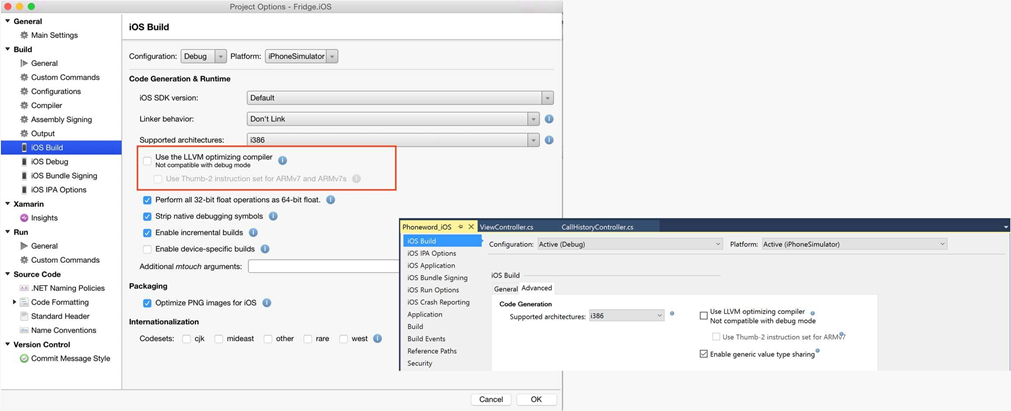
Here some resources:
- Linking on Xamarin.iOS. link
Memory Management
Event Handlers
I know these things are something you’d hear again and again but trust me, it’s very important to remember these rules.
- Always detach Event Handlers and Dispose Observers
- Unsubscribe from events and avoid anonymous delegates to prevent memory leaks
- A reference to the anonymous method can be stored in a field and used to unsubscribe from the event
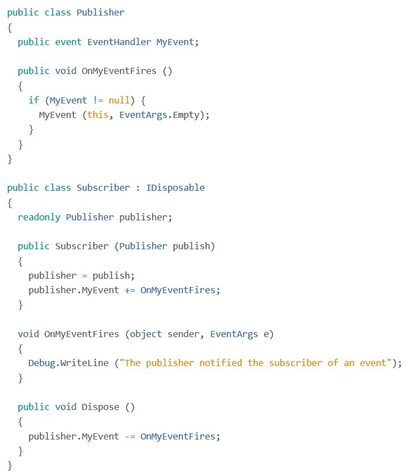
Just remember to unsubscribe from events and remember to unsubscribe them before the subscriber object is disposed of. That’s it, keep that in mind at the beginning.
Later then read the post I’ll give you and apply some other best practices.
Weak references
In .NET, any normal reference to another object is a strong reference. That is, when you declare a variable of a type that’s not a primitive/value type, you are declaring a strong reference.
For example, here object A and object B have strong references to each other, and because of this, this creates what we call an immortal object.
TIP:
Immortal objects are circular strong references. On Xamarin.Android the GC handles that but in Xamarin.iOS we need to be careful.
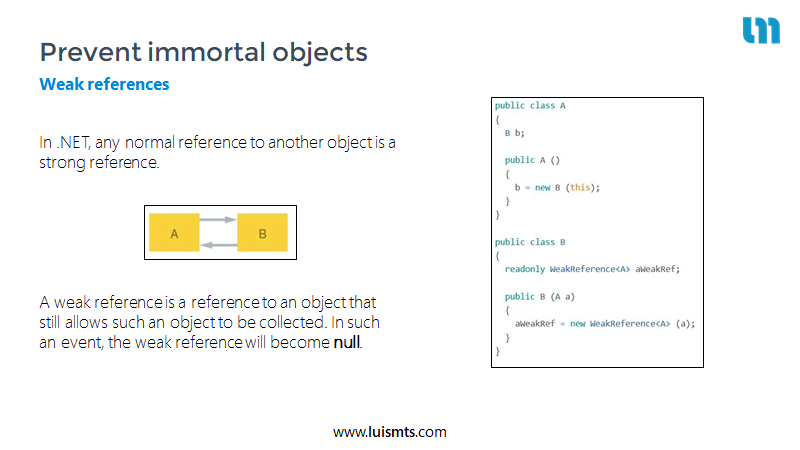
But hey we can Use a weak references to prevent immortal objects.
A weak reference is created using the instance of the object being trapped. So object A maintains a strong reference to object B, but object B maintains a weak reference to Object A.
Load Data Efficiently
When your loading a page you need to think about user experience. You need to be informative when you’re loading your views and their data.
A good practice is to load the local content first, I’m talking about your views and components that you have locally, and in the background then you can start loading your data.
You need to provide to users an experience of like, there’s something happening and not just like there is nothing appearing on the screen.
You can use a placeholder like labels, images, loading icons, activity indicators, etc. Something that I like is defining states like (busy, complete, canceled, etc..) and you can use that with some extra animations.
You need to provide to users an experience of like, there’s something happening and not just like there is nothing appearing on the screen.
You can use a placeholder like labels, images, loading icons, activity indicators, etc. Something that I like is defining states like (busy, complete, canceled, etc..) and you can use that with some extra animations.
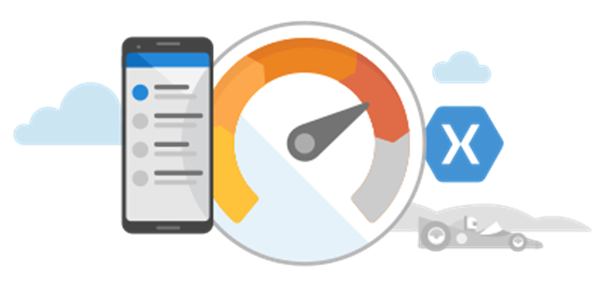