There is a library that is included in all my Xamarin projects and that is Plugin.ValidationRules and more now with its version 1.3 that has a lot of new features to speed up our work. This release has many quality improvements and new features added, including a new Fluent API, method extensions, wrapper for validations with models, among other things.
Why use this solution when there are other more recognized alternatives?
Simply because this library cares about the UI because it’s focused on MVVM. All changes will be reflected in the user interface and you won’t have to create extra properties to handle certain logics. That saves me, and my team, a lot of time.
With nothing else to add. Let’s Start!Contenido
Upgrading to 1.3
This update doesn’t have any drastic changes that force you to change your code when you update, rather it comes with many new features that will help you optimize your time. In short, there’s no abrupt change, if you upgrade now, everything should keep working the same for you.
New features
- HasErrors property added for Validatable objects.
... <Entry Text="{Binding Phone.Value, Mode=TwoWay}" /> <Label Text="{Binding Phone.Error}" IsVisible="{Binding Phone.HasErrors}" /> ...
- Added new Validator Builder for Validatable objects.
- Added new .Build() method to create an empty Validatable object.
- Added new .WithRule(…) method for adding rules to a Validatable object.
- New Condition Method .When(…) added for a Validatable object.
- New Condition Method .Must(…) added for the Validatable object. Now there is no need to create rules if not needed.
public class MyViewModel { public MyViewModel() { ... Phone = Validator.Build<string>() .WithRule(new IsNotNullOrEmptyRule()) .Must(CustomValidation, "Phone number need to be longer.") .When(x => Name.Validate()); } public Validatable<string> Name{ get; set; } public Validatable<string> Phone { get; set; } private bool CustomValidation(string parameter) { return parameter?.Length > 8; } }
- ValidationRule extension methods added:
- New WithMessage added.
- New IsRequired added.
... Phone = Validator.Build<string>() .IsRequired("The phone number is required.") .WithRule(new PhoneNumberRule().WithMessage("This phone number is incorrect."))); ...
- Validator wrapper class to validate aggregated models.
- Create a ValidationUnit with all the Validatable properties of your validator model with the InitUnit() method.
- Generates a default Validate() method that you can use to validate your model validator without moving a finger.
- Use the Build() method to create new Validable objects and their rules with a fluent API.
- Use the Map() method that you can override to map your properties. Remember that in the previous version we added an auto mapper
... public class UserValidator : Validator<User> { public UserValidator2() { //Email validations Email = Build<string>() .IsRequired("A email is required.") .WithRule(new EmailRule()); InitUnit(); } public Validatable<string> Email { get; set; } public override User Map() { // Simple Manual Mapper var manualMapperUser = new User { Email = this.Email.Value }; return manualMapperUser; } } ...
... public UserValidator UserValidator { get; set; } = new(); ... var isValid = UserValidator.Validate(); ... var userData = UserValidator.Map(); ...
ValidationRules by default that you can use with the new Fluent API
Below is the list of default rules in this release:
- IsCreditCard
- IsEmail
- IsEmpty
- IsNotEmpty
- IsEnum
- IsEqual
- IsNotEqual
- IsNotEqual
- IsInclusiveBetween
- IsLessThan
- IsLessThanOrEqual
- IsNull
- IsNotNull
- WithLengthRule
- WithExactLengthRule
- WithMaxLengthRule
- WithMinimumLengthRule
- WithRegularExpression
Extras
One of the advantages of this library is that over time you can create a library of rules that you can reuse in all your projects and thus speed up development times.
When we want to reuse components to the fullest, it is very common to create rules that handle a single task, such as IsNotNullRule. Thinking about this, with complex validation structures we will always have to use several rules in our properties, and most likely we use these rules on multiple pages within our application.
To help developers streamline this process with the new fluent API we can create extensions that add these rules for us. Here’s an example.
... public static Validatable<TModel> IsRequired<TModel>(this Validatable<TModel> validatable, string errorMessage = "") { if(typeof(TModel) == typeof(string)) validatable.Validations.Add(new NotEmptyRule<TModel>("").WithMessage(errorMessage)); if (typeof(TModel).IsClass) validatable.Validations.Add(new NotNullRule<TModel>().WithMessage(errorMessage)); return validatable; } ...
In the end, our fluent API will do all the work for us. Magic!
Resources, Documentation, Examples and Videos
See the release change notes for full details on what’s new in the release. You can also review the initial and complementary documentation that are very useful.
You can also see the documentation from the previous version because it has a lot of new features including the auto mapper for our Model Validators.
I don’t think new documentation is needed, as the new features only support what had already been proposed.
The Xamarin.Forms sample can be reviewed from today and is updated with the new Plugin.ValidationRules version.
Videos
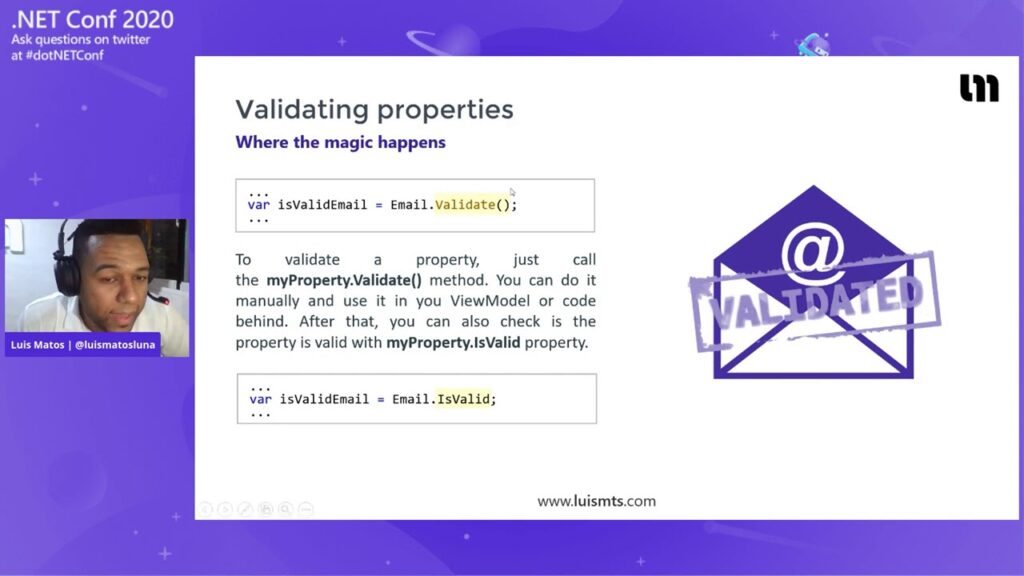
Take a look at this presentation as an initial game to understand what the plugin does. You can find it on YouTube.
conclusion
The truth is that I am very happy with this update, and it is that once you start using the plugin it is very difficult for you to change it or try to use something else.
Also thank Jesse for having the initiative to create a builder and for being a collaborator in this library. Most new features are born from there.
Update the plugin to 1.3 through NuGet Package Manager. Let me know how you’re doing! And if you have any problems, please create an Issue or write to me on Twitter @luismatosluna.